AWS Step Functions – Tutorial
Aws Lambda service offers a convenient way to run your code in the cloud, without worrying too much of launching or managing a server. All this work is done by Aws. It also offers a variety of programming languages like Python, Nodejs, Go, Java, .Net and Ruby.
It’s a breeze to create a new lambda and load your code but what about if you want to create a sequence of lambdas? where the output of one will be the input to the other? and to make it even harder, how about adding logic with “decisions” and “loops“?
Well, in that case a “Step Function” is all what you need. I will try to show you how easy it is to define and execute a step function.
Decision – ChoiceState example
We are going to deploy 4 different lambdas:
– the first will be the “First state”,
– then a ChoiceState will take a decision based on a variable value whether to execute lambda “first choice” or “second choince” and
– finally the last lambda will be “Next state”.
The following step function flow diagram will make things clear:
step function flow diagram
So every time the sequence of lambdas will be [first, firstmatch, next] or [first, secondmatch, next]. Default is a state if none of choices match.
Let’s create these lambdas!
Lambda creation
Lambda1
Go to the console of your AWS account and choose Lambda service. Please select Python language, type the following code:
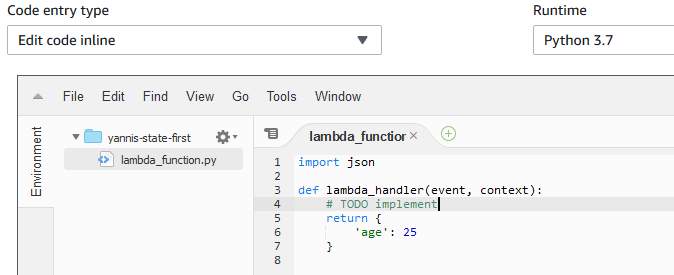
I saved it with the name “yannis-state-first” but you can choose your own one.
Lambda2
Create another lambda and type the following code:
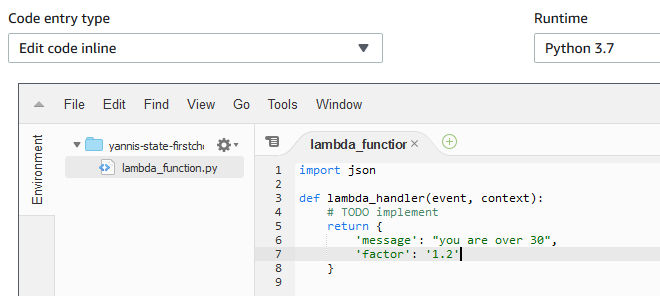
I saved it with the name “yannis-state-firstchoise”.
Lambda3
Create another lambda and type the following code:
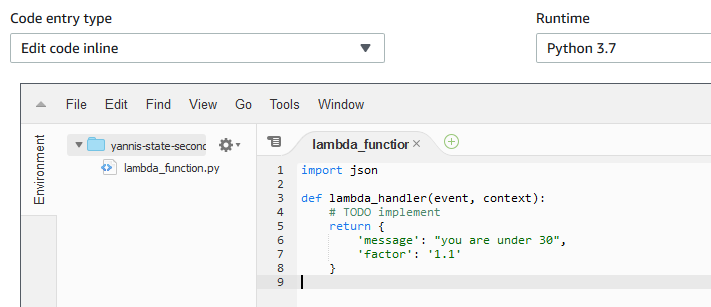
I saved it with the name “yannis-state-secondchoice”.
Lambda4
Finally create a lambda with the following code
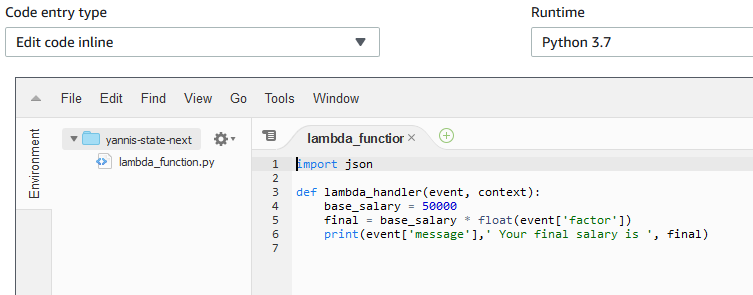
Saved it with the name “yannis-state-next”
Step function definition- JSON
{
"Comment": "An example of the Amazon States Language using a choice state.",
"StartAt": "FirstState",
"States": {
"FirstState": {
"Type": "Task",
"Resource": "arn:aws:lambda:AWS_REGION:AWS_ACCOUNT_ID:function:yannis-state-first",
"Next": "ChoiceState"
},
"ChoiceState": {
"Type" : "Choice",
"Choices": [
{
"Variable": "$.age",
"NumericGreaterThanEquals": 30,
"Next": "FirstMatchState"
},
{
"Variable": "$.age",
"NumericLessThan": 30,
"Next": "SecondMatchState"
}
],
"Default": "DefaultState"
},
"FirstMatchState": {
"Type" : "Task",
"Resource": "arn:aws:lambda:AWS_REGION:AWS_ACCOUNT_ID:function:yannis-state-firstchoise",
"Next": "NextState"
},
"SecondMatchState": {
"Type" : "Task",
"Resource": "arn:aws:lambda:AWS_REGION:AWS_ACCOUNT_ID:function:yannis-state-secondchoice",
"Next": "NextState"
},
"DefaultState": {
"Type": "Fail",
"Error": "DefaultStateError",
"Cause": "No Matches!"
},
"NextState": {
"Type": "Task",
"Resource": "arn:aws:lambda:AWS_REGION:AWS_ACCOUNT_ID:function:yannis-state-next",
"End": true
}
}
}
Explanation
The starting state of our step function is called “FirstState” and has as a resource the arn of the first lambda.
The first lambda returns a respond in JSON format which is:
{
"age": 25
}
This is the input to our next state which is called “ChoiceState” and is actually a decision maker based on the value of “age” variable.
If age >=30 it will execute lambda 2, returning a message that you are over 30 and a variable called “factor” with value “1.2”.
In case age <30 it will execute lambda 3, returning a message and a “factor” with value “1.1”
Finally lambda 4, will calculate the final salary multiplying the “base_salary”=50000 with the factor:
- 1.2 , if age >= 30 or
- 1.1 , if age < 30
Execution
Go to service “Step Function”, give the above JSON definition, replacing the arn of each Lambda (I replaced my AWS_Region and my AWS_Account_Id)
Execute the step function and see the diagram highlights with green colour the path it took:
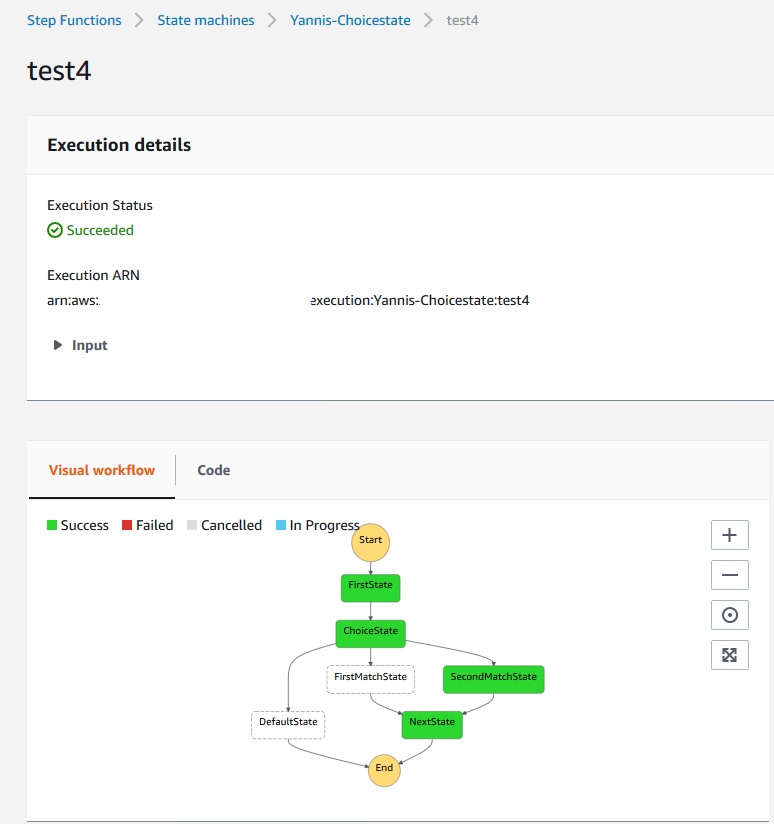
if we change the value of age in the first lambda to be 35 and execute the step function again we will see that it takes another path:
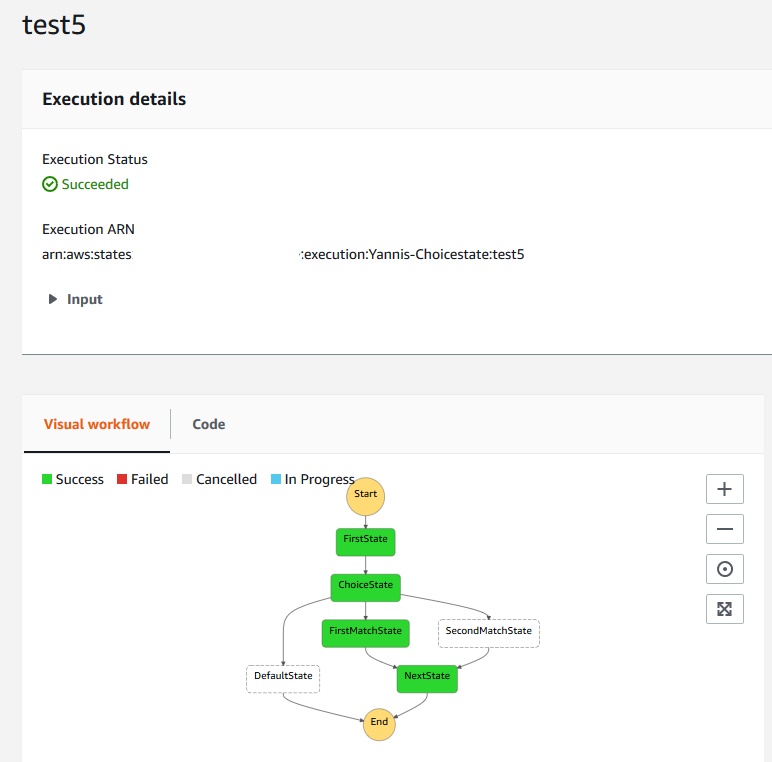
So the Choice State selects the path based on a value of a variable. That is very cool!
if we check the logs in Cloudwatch, we will see the different outputs each time:
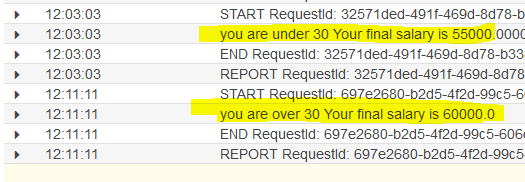
so the final salary will be 55000 if you are under 30, or 60000 if you are over 30.
I hope that I manage to help you build your first step function and understand the logic behind it.
Thank you.